JavaScript
JavaScript is a very powerful client-side
scripting language.
JavaScript is used mainly for enhancing
the interaction of a user with the
webpage.
JavaScript can calculate, manipulate and validate data.
In other words, you can make your
webpage livelier and more interactive, with the help of JavaScript. JavaScript is also being used
widely in game development and Mobile application development.
JavaScript is a scripting language that is used to create and manage dynamic web pages,
basically anything that moves on your screen without requiring you to refresh your browser.
History of javaScript ?
JavaScript was invented by Brendan Eich in 1995. It was developed for Netscape 2, and became the ECMA-262 standard in 1997. JavaScript earlier name is "Mocha" during its development phase at Netscape in 1995. Later on, it was briefly renamed "LiveScript" before ultimately being renamed "JavaScript."
Why JavaScript?
JavaScript offers lots of flexibility.
Mobile app development, desktop app
development, and game development.
With javascript you can find tons of
frameworks and libraries already
developed, which can be used directly in
web development.
Uses Of JavaScript
JavaScript is used in various fields from the
web to servers:
● Web Applications
● Mobile Applications
● Web-based Games
● Back-end Web Development
Features Of JavaScript
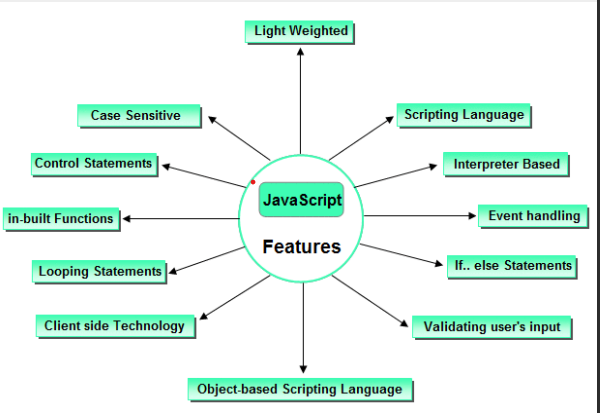
Application of JavaScript
JavaScript is used to create interactive
websites. It is mainly used for:
Client-side validation
Dynamic drop-down menus
Displaying date and time
Displaying pop-up windows and dialog
boxes
Displaying clocks etc
JSS Syntax
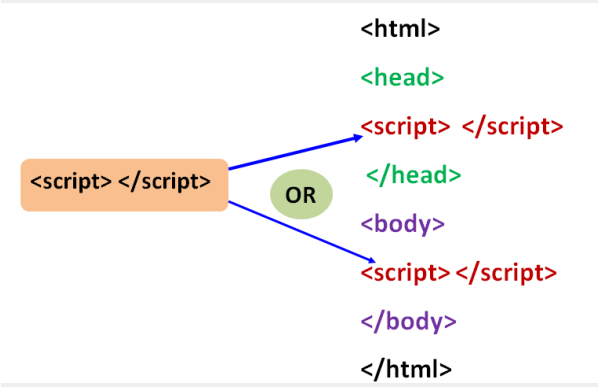
JavaScript Comments
The javascript comments are meaningful way to deliver message.
It is used to add information about the code , warnings or suggestion so that end user can easily interpret the code
Comments are ignored by the browser.
there are two types of comments in js:
Single line comment (//)
Multi-line comment ( /*.........*/)
Places to put JavaScript Code
There are three places to put javascript code:
i. Between the body tag of html
ii. Between the head tag of html
iii. In .js file (external javaScript
— Between the body tag of html —
— Between the head tag of html —
— In .js file (external javaScript —
JavaScript Variable
Variables are used to store data, like string
of text, numbers, etc. The data or value
stored in the variables can be set, updated,
and retrieved whenever needed.
Example:
var name = "Peter Parker";
var age = 21;
var isMarried = false;
JavaScript Datatypes
There are two types of datatypes:
Primitive Types
The primitive data types are the lowest level of the data value in JS.
Numbers : Represents numeric values.
String : Represents sequence of character.
Boolean : Represents boolean value either TRUE or FALSE.
Null : Represents null that is no value at all.
Undefined : Represents undefined value.
Composite/Non-Primitive Types
The non-primitive data types contain some kind of structure with primitive data.
Object : Represents instance through which we can acces member.
Array : Represents groups of similar values.
RegExp : Represents regular Expressions.
JavaScript Operator
There are many types of operator some are:
Arithmetic Operator
Arithmetic operators are used to perform
arithmetic operations on the operands.
Assignment Operator
The following operators are known as
JavaScript assignment operators.
Logical Operator
The following operators are known as
JavaScript logical operators.
Relation or Comparision Operator
The JavaScript comparison operator
compares the two operands.
Conditional Statement
The JavaScript if-else statement is used to execute the code whether condition is true or false.
There are three forms of if statement in JavaScript.
i. If Statement
ii. If else statement
iii. if else if statement
If Statement
It evaluates the content only if expression is true. The signature of JavaScript if statement
is given below.
If else Statement
It evaluates the content whether condition is true of false. The syntax of JavaScript if-else
statement is given below.
If else if Statement
It evaluates the content only if expression is true from several expressions. The signature of
JavaScript if else if statement is given below.
JavaScript Switch
The switch statement is a conditional statement like if statement.
Switch is useful when you wnat to like execute one of the multiple code blocks based on the return value of a specified expression.
JavaScript Loops
The JavaScript loops are used to iterate the piece of code using for, while, do while or for-in
loops. It makes the code compact. It is mostly used in array.
There are four types of loops in JavaScript.
i. for loop
ii. while loop
iii. do-while loop
for loop
The JavaScript for loop iterates the elements for the fixed number of times. It should be used
if number of iterations is known. The syntax of for loop is given below.
while loop
The JavaScript while loop iterates the elements for the infinite number of times. It should be
used if number of iteration is not known. The syntax of while loop is given below.
do-while loop
The JavaScript do while loop iterates the elements for the infinite number of times like while
loop. but, code is executed at least once whether condition is true or false. The syntax of do
while loop is given below
JavaScript Array
JavaScript array is an object that represents a collection of similar type of elements.
There are 3 ways to construct array in JavaScript
i. By array literal by
ii. By creating instance of Array directly (using new keyword)
iii. By using an Array constructor (using new keyword)
By array literal by
The syntax of creating array using array literal is given below:
var arrayname=[value1,value2.....valueN];
By creating instance of Array directly (using new keyword)
The syntax of creating array directly is given below:
var arrayname=new Array();
By using an Array constructor (using new keyword)
Here, you need to create instance of array by passing arguments in constructor so that we don't
have to provide value explicitly.
The example of creating object by array constructor is given below.
JavaScript Object
A JavaScript object is an entity having state and behavior (properties and method). For example:
car, pen, bike, chair, glass, keyboard, monitor etc.
JavaScript is an object-based language. Everything is an object in JavaScript.
JavaScript is template based not class based. Here, we don't create class to get the object. But, we
direct create objects.
There are 3 ways to create objects:
i. By object literal
ii. By creating instance of Object directly (using new keyword)
iii. By using an object constructor (using new keyword)
By object literal
The syntax of creating object using object literal is given below:
object={property1:value1,property2:value2.....propertyN:valueN}
By creating instance of Object directly (using new keyword)
The syntax of creating object directly is given below:
var objectname=new Object();
By using an object constructor (using new keyword)
Here, you need to create function with arguments. Each argument value can be assigned in the
current object by using this keyword.
This keyword refers to the current object.
The example of creating object by object constructor is given below.
JavaScript Function
A function is a block of code that performs a specific task. It takes in input, Processes it, and produces output. Function always returns the value.
Declaring a function
• A function is declared using the function keyword.
• The basic rules of naming a function are similar to naming a variable. It is better to write
a descriptive name for your function. For example, if a function is used to add two
numbers, you could name the function add or addNumbers.
• The body of function is written within {}.